Transaction Flow for MasterCard Level 2/3
This transaction set includes a suite of corporate card financial transactions as well as a transaction that allows for the passing of Level 2/3 data. Please ensure that MasterCard Level 2/3 support is enabled on your merchant account. Batch Close, Open Totals and Pre-Authorization are identical to the non-level 2/3 transactions outlined here.
- When the Pre-Authorization response contains CorporateCard equal to true then you can submit the MasterCard transactions.
- If CorporateCard is false then the card does not support Level 2/3 data, non Level 2/3 transactions are to be used. If the card is not a corporate card, please refer to Non-Level 2/3 transactions for the appropriate non-corporate card transactions.
Note: This transaction set is intended for transactions where Corporate Card is true and Level 2/3 data will be submitted.
If the credit card is found to be a corporate card but you do not wish to send any Level 2/3 data then you may submit MasterCard transactions using the transaction set outlined in Non-Level 2/3 transactions.
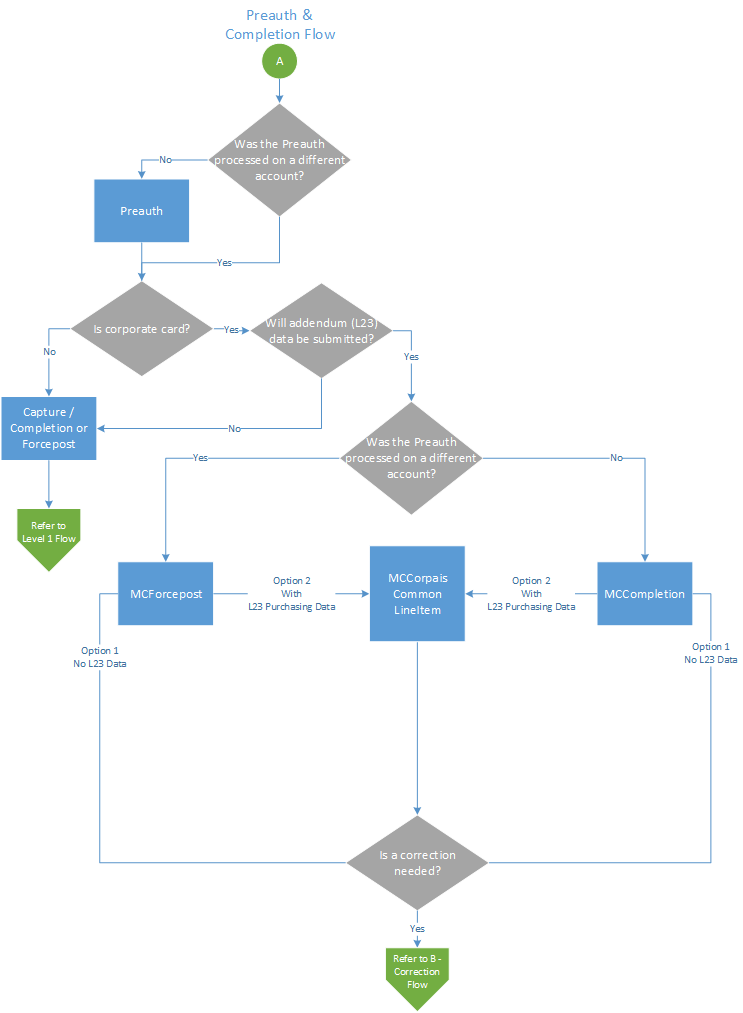
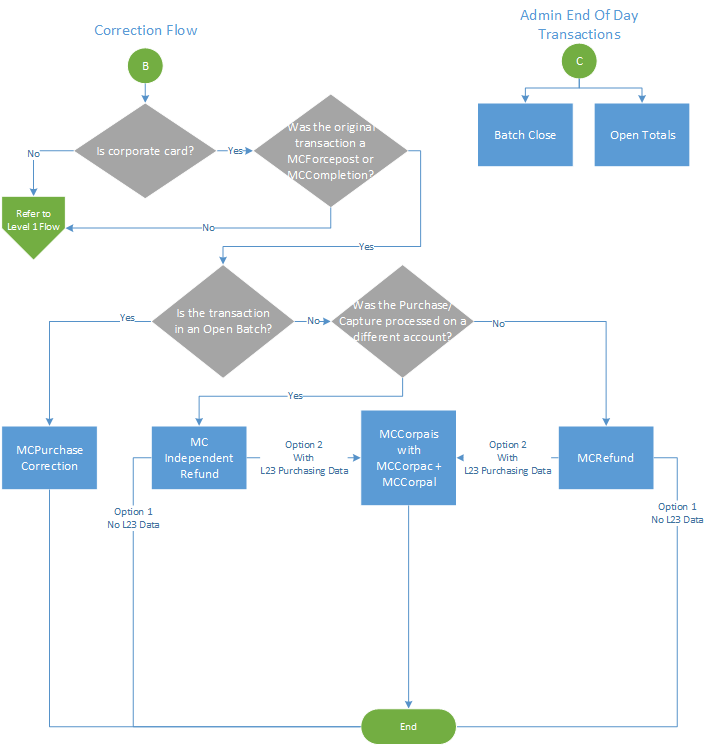
Pre-Authorization
Pre-authorization verifies and locks funds on the customer’s credit card. The funds are locked for a specified amount of time, based on the card issuer. To retrieve the funds from a pre-authorization so that they may be settled in the merchant account a pre-authorization completion must be performed. CorporateCard will return as true if the card supports Level 2/3.
For a list of all possible pre-Authorization options, please refer to this section.
Note: Refer to CorporateCard being returned within the response to determine the next transaction. If the value is”true” proceed with MC Completion. If false, return to Non-Level 2/3 Transactions.
Predecessors:
Successors:
- If CorporateCard is true and Level 2/3 data will be submitted
- Otherwise
- Pre-Authorization Completion ( API | Batch File)
- Vault Tokenize Credit Card ( API )
MC Completion
The MasterCard Completion transaction is used to secure the funds locked by a Pre-Authorization transaction. The pre-authorization completion retrieves the locked funds and readies them for settlement in to the merchant account
Note: Once you have completed this transaction successfully, to submit the complete supplemental level 2/3 data, please proceed to MC Corpais.
package Level23;
import JavaAPI.*;
public class TestMcCompletion
{
public static void main(String[] args)
{
String store_id = "moneris";
String api_token = "hurgle";
String processing_country_code = "CA";
boolean status_check = false;
String order_id="Test1485206444761";
String comp_amount="1.00";
String txn_number="39777-0_11";
String crypt="7";
String merchant_ref_no = "319038";
McCompletion mcCompletion = new McCompletion();
mcCompletion.setOrderId(order_id);
mcCompletion.setCompAmount(comp_amount);
mcCompletion.setTxnNumber(txn_number);
mcCompletion.setCryptType(crypt);
mcCompletion.setMerchantRefNo(merchant_ref_no);
HttpsPostRequest mpgReq = new HttpsPostRequest();
mpgReq.setProcCountryCode(processing_country_code);
mpgReq.setTestMode(true); //false or comment out this line for production transactions
mpgReq.setStoreId(store_id);
mpgReq.setApiToken(api_token);
mpgReq.setTransaction(mcCompletion);
mpgReq.setStatusCheck(status_check);
mpgReq.send();
try
{
Receipt receipt = mpgReq.getReceipt();
System.out.println("CardType = " + receipt.getCardType());
System.out.println("TransAmount = " + receipt.getTransAmount());
System.out.println("TxnNumber = " + receipt.getTxnNumber());
System.out.println("ReceiptId = " + receipt.getReceiptId());
System.out.println("TransType = " + receipt.getTransType());
System.out.println("ReferenceNum = " + receipt.getReferenceNum());
System.out.println("ResponseCode = " + receipt.getResponseCode());
System.out.println("ISO = " + receipt.getISO());
System.out.println("BankTotals = " + receipt.getBankTotals());
System.out.println("Message = " + receipt.getMessage());
System.out.println("AuthCode = " + receipt.getAuthCode());
System.out.println("Complete = " + receipt.getComplete());
System.out.println("TransDate = " + receipt.getTransDate());
System.out.println("TransTime = " + receipt.getTransTime());
System.out.println("Ticket = " + receipt.getTicket());
System.out.println("TimedOut = " + receipt.getTimedOut());
System.out.println("CavvResultCode = " + receipt.getCavvResultCode());
}
catch (Exception e)
{
System.out.println(e);
}
}
}
MCCompletion - Transaction Values
McCompletion mcCompletion = new McCompletion();
HttpsPostRequest mpgReq = new HttpsPostRequest();
mpgReq.setTransaction(mcCompletion);
MCCompletion object mandatory values
Value
|
Type
|
Limits
|
Variable
|
Description
|
Order ID
|
String
|
50-character alphanumeric
|
mcCompletion.setOrderId(order_id);
|
Merchant-defined transaction identifier that must be unique for every Purchase, Pre-Authorization and Independent Refund transaction. No two transactions of these types may have the same order ID.
For Refund, Completion and Purchase Correction transactions, the order ID must be the same as that of the original transaction.
The last 10 characters of the order ID are displayed in the “Invoice Number” field on the Merchant Direct Reports. However only letters, numbers and spaces are sent to Merchant Direct.
A minimum of 3 and a maximum of 10 valid characters are sent to Merchant Direct. Only the last characters beginning after any invalid characters are sent. For example, if the order ID is 1234-567890, only 567890 is sent to Merchant Direct.
If the order ID has fewer than 3 characters, it may display a blank or 0000000000 in the Invoice Number field.
|
Amount
|
String
|
10-character decimal
|
mcCompletion.setCompAmount(comp_amount);
|
Transaction amount This must contain at least 3 digits, two of which are penny values.
The minimum allowable value is $0.01, and the maximum allowable value is $9999999.99. Transaction amounts of $0.00 are not allowed.
|
Transaction Number
|
String
|
255 – character
|
mcCompletion.setTxnNumber(txn_number);
|
Used when performing follow-on transactions. (That is, Completion, Purchase Correction or Refund.) This must be the value that was returned as the transaction number in the response of the original transaction.
When performing a Completion, this value must reference the Pre-Authorization. When performing a Refund or a Purchase Correction, this value must reference the Completion or the Purchase.
|
Merchant Reference Number
|
String
|
19-character alphanumeric
|
mcCompletion.setMerchantRefNo(merchant_ref_no);
|
This data will be displayed in the Merchant Direct Merchant Reference Number field.
|
E-Commerce indicator
|
String
|
1-character alphanumeric
|
mcCompletion.setCryptType(crypt);
|
Describes the category of e-commerce transaction being processed. Allowable values are:
- 1 - Mail Order / Telephone Order—Single
- 2 - Mail Order / Telephone Order—Recurring
- 3 - Mail Order / Telephone Order—Instalment
- 4 - Mail Order / Telephone Order—Unknown classification
- 5 - Authenticated e-commerce transaction (VBV)
- 6 - Non-authenticated e-commerce transaction (VBV)
- 7 - SSL-enabled merchant
In Credential on File transactions where the request field e-commerce indicator is also being sent: the allowable values for e-commerce indicator are dependent on the value sent for payment indicator, as follows:
if payment indicator = R, then allowable values for e-commerce indicator: 2, 5 or 6
if payment indicator = C, then allowable values for e-commerce indicator: 1, 5, 6 or 7
if payment indicator = U, then allowable values for e-commerce indicator: 1 or 7
if payment indicator = Z, then allowable values for e-commerce indicator: 1, 5, 6 or 7
|
Predecessors:
Successors:
MC Forcepost
This transaction is an alternative to MCCompletion to obtain the funds locked on Pre-authorization obtained from IVR or equivalent terminal. The force post requires that the original Pre-authorization’s auth code is provided and it retrieves the locked funds and readies them for settlement in to the merchant account.
Note: Once you have completed this transaction successfully, to submit the complete supplemental level 2/3 data, please proceed to MC Corpais.
package Level23;
import JavaAPI.*;
public class TestMcForcePost
{
public static void main(String[] args)
{
String store_id = "moneris";
String api_token = "hurgle";
String processing_country_code = "CA";
boolean status_check = false;
java.util.Date createDate = new java.util.Date();
String order_id="Test"+createDate.getTime();
String cust_id = "CUST13343";
String amount = "5.00";
String pan = "5454545442424242";
String expiry_date = "1912"; //YYMM
String auth_code = "123456";
String crypt = "7";
String merchant_ref_no = "319038";
McForcePost mcforcepost = new McForcePost();
mcforcepost.setOrderId(order_id);
mcforcepost.setCustId(cust_id);
mcforcepost.setAmount(amount);
mcforcepost.setPan(pan);
mcforcepost.setExpDate(expiry_date);
mcforcepost.setAuthCode(auth_code);
mcforcepost.setCryptType(crypt);
mcforcepost.setMerchantRefNo(merchant_ref_no);
HttpsPostRequest mpgReq = new HttpsPostRequest();
mpgReq.setProcCountryCode(processing_country_code);
mpgReq.setTestMode(true); //false or comment out this line for production transactions
mpgReq.setStoreId(store_id);
mpgReq.setApiToken(api_token);
mpgReq.setTransaction(mcforcepost);
mpgReq.setStatusCheck(status_check);
mpgReq.send();
try
{
Receipt receipt = mpgReq.getReceipt();
System.out.println("CardType = " + receipt.getCardType());
System.out.println("TransAmount = " + receipt.getTransAmount());
System.out.println("TxnNumber = " + receipt.getTxnNumber());
System.out.println("ReceiptId = " + receipt.getReceiptId());
System.out.println("TransType = " + receipt.getTransType());
System.out.println("ReferenceNum = " + receipt.getReferenceNum());
System.out.println("ResponseCode = " + receipt.getResponseCode());
System.out.println("ISO = " + receipt.getISO());
System.out.println("BankTotals = " + receipt.getBankTotals());
System.out.println("Message = " + receipt.getMessage());
System.out.println("AuthCode = " + receipt.getAuthCode());
System.out.println("Complete = " + receipt.getComplete());
System.out.println("TransDate = " + receipt.getTransDate());
System.out.println("TransTime = " + receipt.getTransTime());
System.out.println("Ticket = " + receipt.getTicket());
System.out.println("TimedOut = " + receipt.getTimedOut());
System.out.println("CavvResultCode = " + receipt.getCavvResultCode());
}
catch (Exception e)
{
System.out.println(e);
}
}
}
MC Forcepost - Transaction Values
McForcePost mcforcepost= new McForcePost();
HttpsPostRequest mpgReq = new HttpsPostRequest();
mpgReq.setTransaction(mcforcepost);
MCForcepost object mandatory values
Value
|
Type
|
Limits
|
Variable
|
Description
|
Order ID
|
String
|
50-character alphanumeric
|
mcforcepost.setOrderId(order_id);
|
Merchant-defined transaction identifier that must be unique for every Purchase, Pre-Authorization and Independent Refund transaction. No two transactions of these types may have the same order ID.
For Refund, Completion and Purchase Correction transactions, the order ID must be the same as that of the original transaction.
The last 10 characters of the order ID are displayed in the “Invoice Number” field on the Merchant Direct Reports. However only letters, numbers and spaces are sent to Merchant Direct.
A minimum of 3 and a maximum of 10 valid characters are sent to Merchant Direct. Only the last characters beginning after any invalid characters are sent. For example, if the order ID is 1234-567890, only 567890 is sent to Merchant Direct.
If the order ID has fewer than 3 characters, it may display a blank or 0000000000 in the Invoice Number field.
|
Amount
|
String
|
10-character decimal
|
mcforcepost.setAmount(amount);
|
Transaction amount This must contain at least 3 digits, two of which are penny values.
The minimum allowable value is $0.01, and the maximum allowable value is $9999999.99. Transaction amounts of $0.00 are not allowed.
|
Credit card number
|
String
|
20-character numeric
|
mcforcepost.setPan(pan);
|
Most credit card numbers today are 16 digits, but some 13-digit numbers are still accepted by some issuers. This field has been intentionally expanded to 20 digits in consideration for future expansion and potential support of private label card ranges.
|
Expiry date
|
String
|
4-character alphanumeric (YYMM format)
|
mcforcepost.setExpDate(expiry_date);
|
Note: this is the reverse of the date displayed on the physical card, which is MMYY.
|
Authorization Code
|
String
|
8-character alphanumeric
|
mcforcepost.setAuthCode(auth_code);
|
Authroziation code provided in the transaction response from the issuing bank. This is required for Force Post transactions.
|
Merchant Reference Number
|
String
|
19-character alphanumeric
|
mcforcepost.setMerchantRefNo(merchant_ref_no);
|
This data will be displayed in the Merchant Direct Merchant Reference Number field.
|
E-Commerce indicator
|
String
|
1-character alphanumeric
|
mcforcepost.setCryptType(crypt);
|
Describes the category of e-commerce transaction being processed. Allowable values are:
- 1 - Mail Order / Telephone Order—Single
- 2 - Mail Order / Telephone Order—Recurring
- 3 - Mail Order / Telephone Order—Instalment
- 4 - Mail Order / Telephone Order—Unknown classification
- 5 - Authenticated e-commerce transaction (VBV)
- 6 - Non-authenticated e-commerce transaction (VBV)
- 7 - SSL-enabled merchant
In Credential on File transactions where the request field e-commerce indicator is also being sent: the allowable values for e-commerce indicator are dependent on the value sent for payment indicator, as follows:
if payment indicator = R, then allowable values for e-commerce indicator: 2, 5 or 6
if payment indicator = C, then allowable values for e-commerce indicator: 1, 5, 6 or 7
if payment indicator = U, then allowable values for e-commerce indicator: 1 or 7
if payment indicator = Z, then allowable values for e-commerce indicator: 1, 5, 6 or 7
|
MC Forcepost object optional values
Value
|
Type
|
Limits
|
Variable
|
Description
|
Customer ID
|
String
|
50-character alphanumeric
|
mcforcepost.setCustId(cust_id);
|
This can be used for policy number, membership number, student ID, invoice number and so on.
This field is searchable from the Moneris Merchant Resource Centre.
|
Predecessors:
- Pre-Authorization completed on an independent terminal
Successors:
MC Purchase Correction
MCCompletions can be corrected the same day* that they occur. The MasterCard Purchase Correction (void) transaction is used to cancel a transaction that was performed in the current batch. No amount is required because a purchase correction is always for 100% of the original transaction.
* An MCPurchaseCorrection can be performed against a transaction as long as the batch that contains the original transaction remains open. When using the automated closing feature batch close occurs daily between 10 – 11 pm EST.
package Level23;
import JavaAPI.*;
public class TestMcPurchaseCorrection
{
public static void main(String[] args)
{
String store_id = "moneris";
String api_token = "hurgle";
String processing_country_code = "CA";
boolean status_check = false;
String order_id="Test1485207871499";
String txn_number="660117311902017023164431860-0_11";
String crypt="7";
McPurchaseCorrection mcpurchasecorrection = new McPurchaseCorrection();
mcpurchasecorrection.setOrderId(order_id);
mcpurchasecorrection.setTxnNumber(txn_number);
mcpurchasecorrection.setCryptType(crypt);
HttpsPostRequest mpgReq = new HttpsPostRequest();
mpgReq.setProcCountryCode(processing_country_code);
mpgReq.setTestMode(true); //false or comment out this line for production transactions
mpgReq.setStoreId(store_id);
mpgReq.setApiToken(api_token);
mpgReq.setTransaction(mcpurchasecorrection);
mpgReq.setStatusCheck(status_check);
mpgReq.send();
try
{
Receipt receipt = mpgReq.getReceipt();
System.out.println("CardType = " + receipt.getCardType());
System.out.println("TransAmount = " + receipt.getTransAmount());
System.out.println("TxnNumber = " + receipt.getTxnNumber());
System.out.println("ReceiptId = " + receipt.getReceiptId());
System.out.println("TransType = " + receipt.getTransType());
System.out.println("ReferenceNum = " + receipt.getReferenceNum());
System.out.println("ResponseCode = " + receipt.getResponseCode());
System.out.println("ISO = " + receipt.getISO());
System.out.println("BankTotals = " + receipt.getBankTotals());
System.out.println("Message = " + receipt.getMessage());
System.out.println("AuthCode = " + receipt.getAuthCode());
System.out.println("Complete = " + receipt.getComplete());
System.out.println("TransDate = " + receipt.getTransDate());
System.out.println("TransTime = " + receipt.getTransTime());
System.out.println("Ticket = " + receipt.getTicket());
System.out.println("TimedOut = " + receipt.getTimedOut());
System.out.println("CavvResultCode = " + receipt.getCavvResultCode());
}
catch (Exception e)
{
System.out.println(e);
}
}
}
MCPurchaseCorrection - Transaction Values
McPurchaseCorrection mcpurchasecorrection = new McPurchaseCorrection();
HttpsPostRequest mpgReq = new HttpsPostRequest();
mpgReq.setTransaction(mcpurchasecorrection);
MCPurchaseCorrection object mandatory values
Value
|
Type
|
Limits
|
Variable
|
Description
|
Order ID
|
String
|
50-character alphanumeric
|
mcpurchasecorrection.setOrderId(order_id);
|
Merchant-defined transaction identifier that must be unique for every Purchase, Pre-Authorization and Independent Refund transaction. No two transactions of these types may have the same order ID.
For Refund, Completion and Purchase Correction transactions, the order ID must be the same as that of the original transaction.
The last 10 characters of the order ID are displayed in the “Invoice Number” field on the Merchant Direct Reports. However only letters, numbers and spaces are sent to Merchant Direct.
A minimum of 3 and a maximum of 10 valid characters are sent to Merchant Direct. Only the last characters beginning after any invalid characters are sent. For example, if the order ID is 1234-567890, only 567890 is sent to Merchant Direct.
If the order ID has fewer than 3 characters, it may display a blank or 0000000000 in the Invoice Number field.
|
Transaction Number
|
String
|
255 – character
|
mcpurchasecorrection.setTxnNumber(txn_number);
|
Used when performing follow-on transactions. (That is, Completion, Purchase Correction or Refund.) This must be the value that was returned as the transaction number in the response of the original transaction.
When performing a Completion, this value must reference the Pre-Authorization. When performing a Refund or a Purchase Correction, this value must reference the Completion or the Purchase.
|
E-Commerce indicator
|
String
|
1-character alphanumeric
|
mcpurchasecorrection.setCryptType(crypt);
|
Describes the category of e-commerce transaction being processed. Allowable values are:
- 1 - Mail Order / Telephone Order—Single
- 2 - Mail Order / Telephone Order—Recurring
- 3 - Mail Order / Telephone Order—Instalment
- 4 - Mail Order / Telephone Order—Unknown classification
- 5 - Authenticated e-commerce transaction (VBV)
- 6 - Non-authenticated e-commerce transaction (VBV)
- 7 - SSL-enabled merchant
In Credential on File transactions where the request field e-commerce indicator is also being sent: the allowable values for e-commerce indicator are dependent on the value sent for payment indicator, as follows:
if payment indicator = R, then allowable values for e-commerce indicator: 2, 5 or 6
if payment indicator = C, then allowable values for e-commerce indicator: 1, 5, 6 or 7
if payment indicator = U, then allowable values for e-commerce indicator: 1 or 7
if payment indicator = Z, then allowable values for e-commerce indicator: 1, 5, 6 or 7
|
Predecessors:
Successors:
MC Refund
The MasterCard Refund will credit a specified amount to the cardholder’s credit card. A refund can be sent up to the full value of the original pre-authorization completion.
Note: Once you have completed this transaction successfully, to submit the complete supplemental level 2/3 data, please proceed to MC Corpais.
package Level23;
import JavaAPI.*;
public class TestMcRefund
{
public static void main(String[] args)
{
String store_id = "moneris";
String api_token = "hurgle";
String processing_country_code = "CA";
boolean status_check = false;
String order_id="Test1485207913048";
String amount="5.00";
String txn_number="660117311902017023164513403-0_11";
String crypt="7";
String merchant_ref_no = "319038";
McRefund mcRefund = new McRefund();
mcRefund.setOrderId(order_id);
mcRefund.setAmount(amount);
mcRefund.setTxnNumber(txn_number);
mcRefund.setCryptType(crypt);
mcRefund.setMerchantRefNo(merchant_ref_no);
HttpsPostRequest mpgReq = new HttpsPostRequest();
mpgReq.setProcCountryCode(processing_country_code);
mpgReq.setTestMode(true); //false or comment out this line for production transactions
mpgReq.setStoreId(store_id);
mpgReq.setApiToken(api_token);
mpgReq.setTransaction(mcRefund);
mpgReq.setStatusCheck(status_check);
mpgReq.send();
try
{
Receipt receipt = mpgReq.getReceipt();
System.out.println("CardType = " + receipt.getCardType());
System.out.println("TransAmount = " + receipt.getTransAmount());
System.out.println("TxnNumber = " + receipt.getTxnNumber());
System.out.println("ReceiptId = " + receipt.getReceiptId());
System.out.println("TransType = " + receipt.getTransType());
System.out.println("ReferenceNum = " + receipt.getReferenceNum());
System.out.println("ResponseCode = " + receipt.getResponseCode());
System.out.println("ISO = " + receipt.getISO());
System.out.println("BankTotals = " + receipt.getBankTotals());
System.out.println("Message = " + receipt.getMessage());
System.out.println("AuthCode = " + receipt.getAuthCode());
System.out.println("Complete = " + receipt.getComplete());
System.out.println("TransDate = " + receipt.getTransDate());
System.out.println("TransTime = " + receipt.getTransTime());
System.out.println("Ticket = " + receipt.getTicket());
System.out.println("TimedOut = " + receipt.getTimedOut());
System.out.println("CavvResultCode = " + receipt.getCavvResultCode());
}
catch (Exception e)
{
System.out.println(e);
}
}
}
MCRefund - Transaction Values
McRefund mcRefund = new McRefund();
HttpsPostRequest mpgReq = new HttpsPostRequest();
mpgReq.setTransaction(mcRefund);
MCRefund object mandatory values
Value
|
Type
|
Limits
|
Variable
|
Description
|
Order ID
|
String
|
50-character alphanumeric
|
mcRefund.setOrderId(order_id);
|
Merchant-defined transaction identifier that must be unique for every Purchase, Pre-Authorization and Independent Refund transaction. No two transactions of these types may have the same order ID.
For Refund, Completion and Purchase Correction transactions, the order ID must be the same as that of the original transaction.
The last 10 characters of the order ID are displayed in the “Invoice Number” field on the Merchant Direct Reports. However only letters, numbers and spaces are sent to Merchant Direct.
A minimum of 3 and a maximum of 10 valid characters are sent to Merchant Direct. Only the last characters beginning after any invalid characters are sent. For example, if the order ID is 1234-567890, only 567890 is sent to Merchant Direct.
If the order ID has fewer than 3 characters, it may display a blank or 0000000000 in the Invoice Number field.
|
Amount
|
String
|
10-character decimal
|
mcRefund.setAmount(amount);
|
Transaction amount This must contain at least 3 digits, two of which are penny values.
The minimum allowable value is $0.01, and the maximum allowable value is $9999999.99. Transaction amounts of $0.00 are not allowed.
|
Transaction Number
|
String
|
255 – character
|
mcRefund.setTxnNumber(txn_number);
|
Used when performing follow-on transactions. (That is, Completion, Purchase Correction or Refund.) This must be the value that was returned as the transaction number in the response of the original transaction.
When performing a Completion, this value must reference the Pre-Authorization. When performing a Refund or a Purchase Correction, this value must reference the Completion or the Purchase.
|
Merchant Reference Number
|
String
|
19-character alphanumeric
|
mcRefund.setMerchantRefNo(merchant_ref_no);
|
This data will be displayed in the Merchant Direct Merchant Reference Number field.
|
E-Commerce indicator
|
String
|
1-character alphanumeric
|
mcRefund.setCryptType(crypt);
|
Describes the category of e-commerce transaction being processed. Allowable values are:
- 1 - Mail Order / Telephone Order—Single
- 2 - Mail Order / Telephone Order—Recurring
- 3 - Mail Order / Telephone Order—Instalment
- 4 - Mail Order / Telephone Order—Unknown classification
- 5 - Authenticated e-commerce transaction (VBV)
- 6 - Non-authenticated e-commerce transaction (VBV)
- 7 - SSL-enabled merchant
In Credential on File transactions where the request field e-commerce indicator is also being sent: the allowable values for e-commerce indicator are dependent on the value sent for payment indicator, as follows:
if payment indicator = R, then allowable values for e-commerce indicator: 2, 5 or 6
if payment indicator = C, then allowable values for e-commerce indicator: 1, 5, 6 or 7
if payment indicator = U, then allowable values for e-commerce indicator: 1 or 7
if payment indicator = Z, then allowable values for e-commerce indicator: 1, 5, 6 or 7
|
Predecessors:
Successors:
MC Independent Refund
Independent refund is used when the originating transaction was not performed through Moneris Gateway and does not require an existing order to be logged in the Moneris Gateway; however, the credit card number and the expiry date will need to be passed. The transaction format is almost indentical to a purchse or a pre-authorization.
Please note, the Independent Refund transaction may or may not be supported on your account. If you receive a transaction not allowed error when attempting an independent refund, it may mean the transaction is not supported on your account. If you wish to have the Independent Refund transaction type temporarily enabled (or re-enabled), please contact the Service Centre at 1-866-319-7450.
Note: Once you have completed this transaction successfully, to submit the complete supplemental level 2/3 data, please proceed to MC Corpais.
package Level23;
import JavaAPI.*;
public class TestMcIndependentRefund
{
public static void main(String[] args)
{
String store_id = "moneris";
String api_token = "hurgle";
String processing_country_code = "CA";
boolean status_check = false;
java.util.Date createDate = new java.util.Date();
String order_id="Test"+createDate.getTime();
String cust_id = "CUST13343";
String amount = "5.00";
String pan = "5454545442424242";
String expiry_date = "1912"; //YYMM
String crypt = "7";
String merchant_ref_no = "319038";
McIndependentRefund mcindrefund = new McIndependentRefund();
mcindrefund.setOrderId(order_id);
mcindrefund.setCustId(cust_id);
mcindrefund.setAmount(amount);
mcindrefund.setPan(pan);
mcindrefund.setExpDate(expiry_date);
mcindrefund.setCryptType(crypt);
mcindrefund.setMerchantRefNo(merchant_ref_no);
HttpsPostRequest mpgReq = new HttpsPostRequest();
mpgReq.setProcCountryCode(processing_country_code);
mpgReq.setTestMode(true); //false or comment out this line for production transactions
mpgReq.setStoreId(store_id);
mpgReq.setApiToken(api_token);
mpgReq.setTransaction(mcindrefund);
mpgReq.setStatusCheck(status_check);
mpgReq.send();
try
{
Receipt receipt = mpgReq.getReceipt();
System.out.println("CardType = " + receipt.getCardType());
System.out.println("TransAmount = " + receipt.getTransAmount());
System.out.println("TxnNumber = " + receipt.getTxnNumber());
System.out.println("ReceiptId = " + receipt.getReceiptId());
System.out.println("TransType = " + receipt.getTransType());
System.out.println("ReferenceNum = " + receipt.getReferenceNum());
System.out.println("ResponseCode = " + receipt.getResponseCode());
System.out.println("ISO = " + receipt.getISO());
System.out.println("BankTotals = " + receipt.getBankTotals());
System.out.println("Message = " + receipt.getMessage());
System.out.println("AuthCode = " + receipt.getAuthCode());
System.out.println("Complete = " + receipt.getComplete());
System.out.println("TransDate = " + receipt.getTransDate());
System.out.println("TransTime = " + receipt.getTransTime());
System.out.println("Ticket = " + receipt.getTicket());
System.out.println("TimedOut = " + receipt.getTimedOut());
System.out.println("CavvResultCode = " + receipt.getCavvResultCode());
}
catch (Exception e)
{
System.out.println(e);
}
}
}
MCIndependent Refund - Transaction Values
McIndependentRefund mcindrefund = new McIndependentRefund();
HttpsPostRequest mpgReq = new HttpsPostRequest();
mpgReq.setTransaction(mcindrefund);
MCIndependent Refund object mandatory values
Value
|
Type
|
Limits
|
Variable
|
Description
|
Order ID
|
String
|
50-character alphanumeric
|
mcindrefund.setOrderId(order_id);
|
Merchant-defined transaction identifier that must be unique for every Purchase, Pre-Authorization and Independent Refund transaction. No two transactions of these types may have the same order ID.
For Refund, Completion and Purchase Correction transactions, the order ID must be the same as that of the original transaction.
The last 10 characters of the order ID are displayed in the “Invoice Number” field on the Merchant Direct Reports. However only letters, numbers and spaces are sent to Merchant Direct.
A minimum of 3 and a maximum of 10 valid characters are sent to Merchant Direct. Only the last characters beginning after any invalid characters are sent. For example, if the order ID is 1234-567890, only 567890 is sent to Merchant Direct.
If the order ID has fewer than 3 characters, it may display a blank or 0000000000 in the Invoice Number field.
|
Amount
|
String
|
10-character decimal
|
mcindrefund.setAmount(amount);
|
Transaction amount This must contain at least 3 digits, two of which are penny values.
The minimum allowable value is $0.01, and the maximum allowable value is $9999999.99. Transaction amounts of $0.00 are not allowed.
|
Credit card number
|
String
|
20-character numeric
|
mcindrefund.setPan(pan);
|
Most credit card numbers today are 16 digits, but some 13-digit numbers are still accepted by some issuers. This field has been intentionally expanded to 20 digits in consideration for future expansion and potential support of private label card ranges.
|
Expiry date
|
String
|
4-character numeric
YYMM format.
|
mcindrefund.setExpDate(expiry_date);
|
Submit in YYMM format.
Note: This is the reverse of the date displayed on the physical card, which is MMYY.
|
Merchant Reference Number
|
String
|
19-character alphanumeric
|
mcindrefund.setMerchantRefNo(merchant_ref_no);
|
This data will be displayed in the Merchant Direct Merchant Reference Number field.
|
E-Commerce indicator
|
String
|
1-character alphanumeric
|
mcindrefund.setCryptType(crypt);
|
Describes the category of e-commerce transaction being processed. Allowable values are:
- 1 - Mail Order / Telephone Order—Single
- 2 - Mail Order / Telephone Order—Recurring
- 3 - Mail Order / Telephone Order—Instalment
- 4 - Mail Order / Telephone Order—Unknown classification
- 5 - Authenticated e-commerce transaction (VBV)
- 6 - Non-authenticated e-commerce transaction (VBV)
- 7 - SSL-enabled merchant
In Credential on File transactions where the request field e-commerce indicator is also being sent: the allowable values for e-commerce indicator are dependent on the value sent for payment indicator, as follows:
if payment indicator = R, then allowable values for e-commerce indicator: 2, 5 or 6
if payment indicator = C, then allowable values for e-commerce indicator: 1, 5, 6 or 7
if payment indicator = U, then allowable values for e-commerce indicator: 1 or 7
if payment indicator = Z, then allowable values for e-commerce indicator: 1, 5, 6 or 7
|
MCIndependentRefund object optional values
Value
|
Type
|
Limits
|
Variable
|
Description
|
Customer ID
|
String
|
50-character alphanumeric
|
mcindrefund.setCustId(cust_id);
|
This can be used for policy number, membership number, student ID, invoice number and so on.
This field is searchable from the Moneris Merchant Resource Centre.
|
Predecessors:
- Transaction that was completed on an independent system
Successors:
MC Corpais - Corporate Card Common Data with Line Item Details
This transaction example includes the following elements for Level 2 and 3 purchasing card corporate card data processing:
- Corporate Card Common Data (MCCorpac)
- only 1 set of MCCorpac fields can be submitted
- this data set includes data elements that apply to the overall order, for example the total overall taxes
- Line Item Details (MCCorpal)
- 1-998 counts of MCCorpal line items can be submitted
- This data set includes the details about each individual item or service purchased
MCCorpaisCommonLineItem request must be preceded by a financial transaction (MCCompletion,
MCForcepost, MCRefund, MCIndependentRefund) and the Corporate Card flag must be set to “true” in
the Preauth response. MCCorpaisCommonLineItem request will need to contain the order_id of the financial
transaction as well the txn_number.
package Level23;
import JavaAPI.*;
public class TestMcCorpaisCommonLineItem
{
public static void main(String[] args)
{
String store_id = "moneris";
String api_token = "hurgle";
String processing_country_code = "CA";
boolean status_check = false;
String order_id="Test1485206444761";
String txn_number="39777-1_11";
String customer_code1_c ="CustomerCode123";
String card_acceptor_tax_id_c ="UrTaxId";//Merchant tax id which is mandatory
String corporation_vat_number_c ="cvn123";
String freight_amount_c ="1.23";
String duty_amount_c ="2.34";
String ship_to_pos_code_c ="M1R 1W5";
String order_date_c ="141211";
String customer_vat_number_c ="customervn231";
String unique_invoice_number_c ="uin567";
String authorized_contact_name_c ="John Walker";
//Tax Details
String[] tax_amount_c = { "1.19", "1.29"};
String[] tax_rate_c = { "6.0", "7.0"};
String[] tax_type_c = { "GST", "PST"};
String[] tax_id_c = { "gst1298", "pst1298"};
String[] tax_included_in_sales_c = { "Y", "N"};
//Item Details
String[] customer_code1_l = {"customer code", "customer code2"};
String[] line_item_date_l = {"150114", "150114"};
String[] ship_date_l = {"150120", "150122"};
String[] order_date1_l = {"150114", "150114"};
String[] medical_services_ship_to_health_industry_number_l = {"", ""};
String[] contract_number_l = {"", ""};
String[] medical_services_adjustment_l = {"", ""};
String[] medical_services_product_number_qualifier_l = {"", ""};
String[] product_code1_l = {"pc11", "pc12"};
String[] item_description_l = {"Good item", "Better item"};
String[] item_quantity_l = {"4", "5"};
String[] unit_cost_l ={"1.25", "10.00"};
String[] item_unit_measure_l = {"EA", "EA"};
String[] ext_item_amount_l ={"5.00", "50.00"};
String[] discount_amount_l ={"1.00", "50.00"};
String[] commodity_code_l ={"cCode11", "cCode12"};
String[] type_of_supply_l = {"", ""};
String[] vat_ref_num_l = {"", ""};
//Tax Details for Items
String[] tax_amount_l = {"0.52", "1.48"};
String[] tax_rate_l = {"13.0", "13.0"};
String[] tax_type_l = {"HST", "HST"};
String[] tax_id_l = {"hst1298", "hst1298"};
String[] tax_included_in_sales_l = {"Y", "Y"};
//Create and set Tax for McCorpac
McTax tax_c = new McTax();
tax_c.setTax(tax_amount_c[0], tax_rate_c[0], tax_type_c[0], tax_id_c[0], tax_included_in_sales_c[0]);
tax_c.setTax(tax_amount_c[1], tax_rate_c[1], tax_type_c[1], tax_id_c[1], tax_included_in_sales_c[1]);
//Create and set McCorpac for common data - only set values that you know
McCorpac mcCorpac = new McCorpac();
mcCorpac.setCustomerCode1(customer_code1_c);
mcCorpac.setCardAcceptorTaxTd(card_acceptor_tax_id_c);
mcCorpac.setCorporationVatNumber(corporation_vat_number_c);
mcCorpac.setFreightAmount1(freight_amount_c);
mcCorpac.setDutyAmount1(duty_amount_c);
mcCorpac.setShipToPosCode(ship_to_pos_code_c);
mcCorpac.setOrderDate(order_date_c);
mcCorpac.setCustomerVatNumber(customer_vat_number_c);
mcCorpac.setUniqueInvoiceNumber(unique_invoice_number_c);
mcCorpac.setAuthorizedContactName(authorized_contact_name_c);
mcCorpac.setTax(tax_c);
//Create and set Tax for McCorpal
McTax[] tax_l = new McTax[2];
tax_l[0] = new McTax();
tax_l[0].setTax(tax_amount_l[0], tax_rate_l[0], tax_type_l[0], tax_id_l[0], tax_included_in_sales_l[0]);
tax_l[1] = new McTax();
tax_l[1].setTax(tax_amount_l[1], tax_rate_l[1], tax_type_l[1], tax_id_l[1], tax_included_in_sales_l[1]);
//Create and set McCorpal for each item
McCorpal mcCorpal = new McCorpal();
mcCorpal.setMcCorpal(customer_code1_l[0], line_item_date_l[0], ship_date_l[0], order_date1_l[0], medical_services_ship_to_health_industry_number_l[0], contract_number_l[0],
medical_services_adjustment_l[0], medical_services_product_number_qualifier_l[0], product_code1_l[0], item_description_l[0], item_quantity_l[0],
unit_cost_l[0], item_unit_measure_l[0], ext_item_amount_l[0], discount_amount_l[0], commodity_code_l[0], type_of_supply_l[0], vat_ref_num_l[0], tax_l[0]);
mcCorpal.setMcCorpal(customer_code1_l[1], line_item_date_l[1], ship_date_l[1], order_date1_l[1], medical_services_ship_to_health_industry_number_l[1], contract_number_l[1],
medical_services_adjustment_l[1], medical_services_product_number_qualifier_l[1], product_code1_l[1], item_description_l[1], item_quantity_l[1],
unit_cost_l[1], item_unit_measure_l[1], ext_item_amount_l[1], discount_amount_l[1], commodity_code_l[1], type_of_supply_l[1], vat_ref_num_l[1], tax_l[1]);
McCorpais mcCorpais = new McCorpais();
mcCorpais.setOrderId(order_id);
mcCorpais.setTxnNumber(txn_number);
mcCorpais.setMcCorpac(mcCorpac);
mcCorpais.setMcCorpal(mcCorpal);
HttpsPostRequest mpgReq = new HttpsPostRequest();
mpgReq.setProcCountryCode(processing_country_code);
mpgReq.setTestMode(true); //false or comment out this line for production transactions
mpgReq.setStoreId(store_id);
mpgReq.setApiToken(api_token);
mpgReq.setTransaction(mcCorpais);
mpgReq.setStatusCheck(status_check);
mpgReq.send();
try
{
Receipt receipt = mpgReq.getReceipt();
System.out.println("CardType = " + receipt.getCardType());
System.out.println("TransAmount = " + receipt.getTransAmount());
System.out.println("TxnNumber = " + receipt.getTxnNumber());
System.out.println("ReceiptId = " + receipt.getReceiptId());
System.out.println("TransType = " + receipt.getTransType());
System.out.println("ReferenceNum = " + receipt.getReferenceNum());
System.out.println("ResponseCode = " + receipt.getResponseCode());
System.out.println("ISO = " + receipt.getISO());
System.out.println("BankTotals = " + receipt.getBankTotals());
System.out.println("Message = " + receipt.getMessage());
System.out.println("AuthCode = " + receipt.getAuthCode());
System.out.println("Complete = " + receipt.getComplete());
System.out.println("TransDate = " + receipt.getTransDate());
System.out.println("TransTime = " + receipt.getTransTime());
System.out.println("Ticket = " + receipt.getTicket());
System.out.println("TimedOut = " + receipt.getTimedOut());
System.out.println("CavvResultCode = " + receipt.getCavvResultCode());
}
catch (Exception e)
{
System.out.println(e);
}
}
}
MC Corpais – Corporate Card Common Data with Line Item Details - Transaction Values
McCorpais mcCorpais = new McCorpais();
HttpsPostRequest mpgReq = new HttpsPostRequest();
mpgReq.setTransaction(mcCorpais);
MC Corpais – Corporate Card Common Data with Line Item Details mandatory values
MasterCard - Purchasing Corporate Card Data Processing - Level 2 Request Fields
Corporate Card Common Data (MCCorpac)
MasterCard - Purchasing Corporate Card Data Processing - Level 3 Request Fields
Line Item Details (MCCorpal)
mcCorpal.setMcCorpal(customer_code1_l[0], line_item_date_l[0], ship_date_l[0], order_date1_l[0], medical_services_ship_to_health_industry_number_l[0], contract_number_l[0],medical_services_adjustment_l[0], medical_services_product_number_qualifier_l[0], product_code1_l[0], item_description_l[0], item_quantity_l[0], unit_cost_l[0], item_unit_measure_l[0], ext_item_amount_l[0], discount_amount_l[0], commodity_code_l[0], type_of_supply_l[0], vat_ref_num_l[0], tax_l[0]);
Tax Array Request Fields
Setting tax array for MC Corpac
//Tax Details
string[] tax_amount_c = { "1.19", "1.29"};
string[] tax_rate_c = { "6.0", "7.0"};
string[] tax_type_c = { "GST", "PST"};
string[] tax_id_c = { "gst1298", "pst1298"};
string[] tax_included_in_sales_c = { "Y", "N"};
McTax tax_c = new McTax();
tax_c.setTax(tax_amount_c[0], tax_rate_c[0], tax_type_c[0], tax_id_c[0], tax_included_in_sales_c[0]);
Setting tax array for Mc Corpal
//Tax Details for Items
string[] tax_amount_l = {"0.52", "1.48"};
string[] tax_rate_l = {"13.0", "13.0"};
string[] tax_type_l = {"HST", "HST"};
string[] tax_id_l = {"hst1298", "hst1298"};
string[] tax_included_in_sales_l = {"Y", "Y"};
McTax[] tax_l = new McTax[2];
tax_l[1].setTax(tax_amount_l[1], tax_rate_l[1], tax_type_l[1], tax_id_l[1], tax_included_in_sales_l[1]);
Predecessors:
Successors:
Response Fields
Please refer to Response Fields.